Multi-select values (choice or person fields where you can pick more than one) have traditionally been tough to work with in List Formatting. You can use the values directly but right away you’ll see formatting differences from the standard/default formatting:
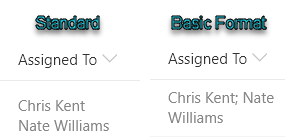
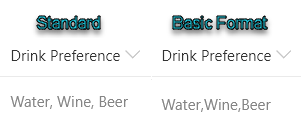
Person fields get separated by a semi-colon and a space, choice values are separated by a comma and lose the space. Aw, come on!
Fortunately, you can have some control over how these are separated using the join function. The join function takes in an array as the first parameter and the separator text to use between items. Here are some samples:
Expression | Field Value | Result |
“=join(@currentField, ‘, ‘)” | [Water,Wine,Beer] | Water, Wine, Beer |
“=join(@currentField, ‘|’)” | [Water,Wine,Beer] | Water|Wine|Beer |
“=join(@currentField, ‘ & ‘)” | [Water,Wine,Beer] | Water & Wine & Beer |
That’s pretty slick!
If you read my last post about using the indexOf function to see if your text contains a given value you might think you could use this to see if the selected values contain one of the choices/person fields. Not quite…
Selection Contains a Value
The indexOf function works on strings not arrays. Multi-select fields are arrays internally and the indexOf function will always return -1 when applied directly to the array value.
Fortunately, we can either use the join function shown above or the toString function on our array value first, then we can easily apply our contains logic using the indexOf function!
I recommend using the join function so that you know exactly what you’re going to get since the toString will vary it’s separator depending on the field type (choice or person).
Here are some examples of combining these functions to see if a choice is selected:
Expression | Field Value | Result |
“=if(indexOf(join(@currentField,”),’Egg’) != -1, ‘Yes’,’No’)” | [Egg,Hat] | Yes |
“=if(indexOf(join(@currentField,”),’Hat’) != -1, ‘Yes’,’No’)” | [Egg,Hat] | Yes |
“=if(indexOf(join(@currentField,”),’Egg’) != -1, ‘Yes’,’No’)” | [Hat,Dog] | No |
We can use this technique within our formats to create some very interesting visualizations:
{ "$schema": "https://developer.microsoft.com/json-schemas/sp/column-formatting.schema.json", "elmType": "div", "style": { "font-size": "16px" }, "children": [ { "elmType": "span", "attributes": { "title": "Water", "iconName": "Precipitation", "class": "='ms-fontColor-' + if(indexOf(join(@currentField,''),'Water') != -1, 'themeDark', 'neutralLight')" }, "style": { "padding": "0 2px" } }, { "elmType": "span", "attributes": { "title": "Coffee", "iconName": "CoffeeScript", "class": "='ms-fontColor-' + if(indexOf(join(@currentField,''),'Coffee') != -1, 'themeDark', 'neutralLight')" }, "style": { "padding": "0 2px 0 0" } }, { "elmType": "span", "attributes": { "title": "Wine", "iconName": "Wines", "class": "='ms-fontColor-' + if(indexOf(join(@currentField,''),'Wine') != -1, 'themeDark', 'neutralLight')" }, "style": { "padding": "0 2px" } }, { "elmType": "span", "attributes": { "title": "Beer", "iconName": "BeerMug", "class": "='ms-fontColor-' + if(indexOf(join(@currentField,''),'Beer') != -1, 'themeDark', 'neutralLight')" }, "style": { "padding": "0 2px" } }, { "elmType": "span", "attributes": { "title": "\"Juice\"", "iconName": "TestBeaker", "class": "='ms-fontColor-' + if(indexOf(join(@currentField,''),'\"Juice\"') != -1, 'themeDark', 'neutralLight')" }, "style": { "padding": "0 2px" } } ] }
PnP Sample: multi-choice-icons
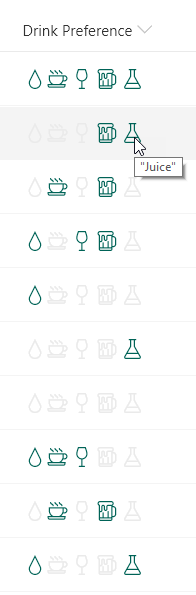
Now you can easily work with multi-select fields and apply dynamic formats that can take these fields from strings of text to meaningful visualizations.
Love List Formatting?
Join the Bi-weekly (every other Thursday) SharePoint Patterns and Practices special interest group for general development call where I will be presenting a new List Formatting Quick Tip on each call!
Also, come get the full picture in my sessions about List Formatting at the SharePoint Conference in Las Vegas in May, or the European Collaboration Summit in Germany in May:
- Use KENT to save $50 for the SharePoint Conference
- Register for the European Collaboration Summit